Notification Types Available
- Basic notification
- Big picture notification
- Media notification
- Big Text notification
- Inbox notification
- Messaging notification
- Messaging Group notification
- Notifications with action buttons
- Grouped notifications
- Progress bar notifications (only for Android)
All notifications could be created locally or via Firebase services, with all the features.
Initial Configurations
Bellow are the obligatory configurations that your app must meet to use awesome_notifications:
???? Configuring Android
1 – Is required the minimum android SDK to 21 (Android 5.0 Lollipop) and Java compiled SDK Version to 33 (Android 13). You can change the minSdkVersion
to 21 and the compileSdkVersion
and targetSdkVersion
to 33, inside the file build.gradle
, located inside “android/app/” folder.
android {
compileSdkVersion 33
defaultConfig {
minSdkVersion 21
targetSdkVersion 33
...
}
...
}
DartAlso, to turn your app fully compatible with Android 13 (SDK 33), you need to add the attribute android:exported="true"
to any <activity>, <activity-alias>, <service>, or <receiver> components that have <intent-filter> declared inside in the app’s AndroidManifest.xml file, and that’s turns required for every other flutter packages that you’re using.
<manifest xmlns:android="http://schemas.android.com/apk/res/android"
package="com.example.myapp">
<application>
...
<activity
android:name=".MainActivity"
...
android:exported="true">
...
</activity>
...
</application>
</manifest>
Dart???? Configuring iOS
To use Awesome Notifications and build your app correctly, you need to ensure to set some build settings
options for your app targets. In your project view, click on Runner -> Target Runner -> Build settings…
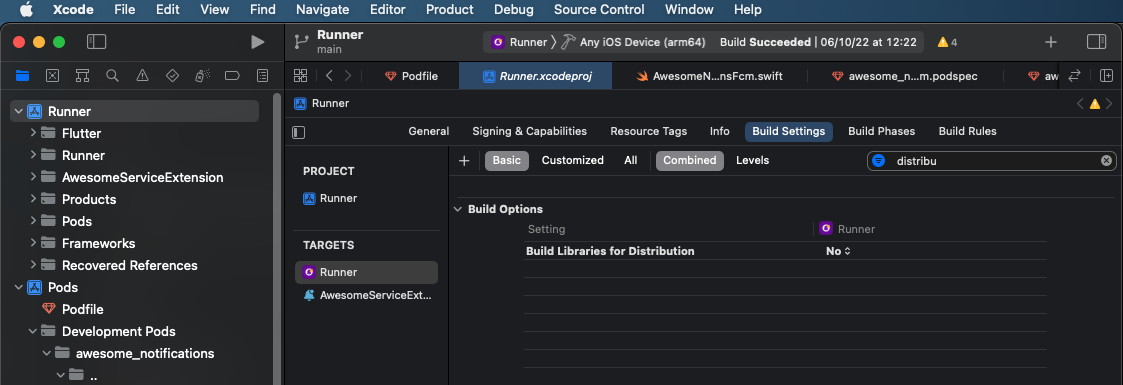
… and set the following options:
In Runner Target:
- Build libraries for distribution => NO
- Only safe API extensions => NO
- iOS Deployment Target => 11 or greater
In all other Targets:
- Build libraries for distribution => NO
- Only safe API extensions => YES
How to show Local Notifications
- Add awesome_notifications as a dependency in your
pubspec.yaml
file.
awesome_notifications
: ^0.7.3
- import the plugin package to your dart code
import’package:awesome_notifications/awesome_notifications.dart’
;
- Initialize the plugin on main.dart, before MaterialApp widget (preferentially inside main() method), with at least one native icon and one channel
main.dart
void main() async {
await ScreenUtil.ensureScreenSize();
HttpOverrides.global = new MyHttpOverrides();
WidgetsFlutterBinding.ensureInitialized();
await Firebase.initializeApp();
//initialMessage = await FirebaseMessaging.instance.getInitialMessage();
AwesomeNotifications().initialize(
null,
[
NotificationChannel(
channelKey: 'basic_channel',
channelName: 'Basic notifications',
channelDescription: 'Notification channel for basic tests',
defaultColor: Color(0xFF9D50DD),
importance: NotificationImportance.High,
channelShowBadge: true,
ledColor: Colors.white)
],
channelGroups: [
NotificationChannelGroup(
channelGroupKey: 'basic_channel_group',
channelGroupName: 'Basic group'),
],
debug: true);
await FirebaseMessaging.instance.setForegroundNotificationPresentationOptions(
alert: true, badge: true, sound: true);
await FlutterDownloader.initialize(
debug:
true, // optional: set to false to disable printing logs to console (default: true)
ignoreSsl:
true // option: set to false to disable working with http links (default: false)
);
runApp(const MyApp());
}
Dart4. In any place of your app, create a new notification
Any Screen
FirebaseMessaging.onMessage.listen((RemoteMessage remotemessage) {
RemoteNotification? notification = remotemessage.notification;
AndroidNotification? android = remotemessage.notification?.android;
if (notification != null && android != null) {
AwesomeNotifications().createNotification(
content: NotificationContent(
id: 1,
channelKey: 'basic_channel',
title: notification.title,
body: notification.body,
showWhen: true,
displayOnForeground: true,
displayOnBackground: true,
));
}
});
FirebaseMessaging.onMessageOpenedApp.listen((RemoteMessage message) async {
RemoteNotification? notification = message.notification;
AndroidNotification? android = message.notification?.android;
if (notification != null && android != null) {
AwesomeNotifications().createNotification(
content: NotificationContent(
id: 1,
channelKey: 'basic_channel',
title: notification.title,
body: notification.body,
showWhen: true,
displayOnForeground: true,
displayOnBackground: true,
bigPicture: notification.android!.imageUrl));
}
});
DartCopy???????????? THATS IT! CONGRATZ MY FRIEND!!! ????????????
Copy